ROS Topic
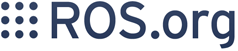
Notes about an ROS Topic. This will describe a basic publisher and subscriber topic. Where to find the list of topics and the list of possible messages for a topic. How to install a new message for a topic.
rostopic list
rosmsg list
rostopic type <topic>
rostopic echo <topic>
rostopic info <topic>
rostopic hz <topic>
Publisher
Publish messages into a topic
Publish in Code
import rospy
from geometry_msgs.msg import Twist
rospy.init_node('robot_mover') # Create Publisher
pub = rospy.Publisher('/cmd_move', Twist, queue_size=1) # Set the data type for the publisher
rate = rospy.Rate(2) # 2hz
move = Twist() # Create a Twist object
move.linear.x = 0.5 # Move forward on x 0.5
move.angular.z = 0.5 # Move at an anglular rate of 0.5
while not rospy.is_shutdown():
pub.publish(move) # Publish the move
rate.sleep() # Maintain the refresh rate
Publish on Commandline
Manually publish in command line
rostopic pub /cmd_move geometry_msgs/Twist "linear: x: 0.5 y: 0.0 z: 0.0 angular: x: 0.0 y: 0.0 z: 0.5"
rostopic pub <topic_name> <message_type> <value>
You can use the ‘double tab’ to autofil the value.
Subscriber
Read messages from a topic
Subscribe in Code
import rospy
from std_msgs.msg import Int32
def callback(msg)
print(msg.data)
rospy.init_node('topic_subscriber')
sub = rospy.Subscriber('/counter', Int32, callback)
rospy.spin()
Determine the message type of the topic
rostopic info /counter
This will return back the data type, publishers and subscribers for the given topic.
Create a Custom Data Type
Create a directory in the package
roscd my_new_package
mkdir msg
Create the Data type
Create a file within the new directory for the new data type Call it Vector.msg. Add the following in to the file
float32 magnitude
float32 direction
Edit CMakeLists.txt
Edit CMakeLists.txt in the package to find the new data type. Look for find_package(catkin REQUIRED COMPONENTS …)
find_package(catkin REQUIRED COMPONENTS
rospy
std_msgs
message_generation
)
Again within CMakeLists.txt search for catkin_package(…) and uncomment the following line and append it if it contains other things passed CAKTIN_DEPENDS.
catkin_package(
CATKIN_DEPENDS message_runtime
)
Again within CMakeLists.txt search for add_message_files(…) and uncomment it and add the new messages.
add_message_files(
FILES
Vector.msg
)
Again within CMakeLists.txt search for generate_messages(…) and uncomment it.
generate_messages(
DEPENDENCIES
std_msgs
)
Save the CMakeLists.txt file.
Edit package.xml
Edit package.xml in the package to find our new data type. Find build_depend…
<build_depend>message_generation</build_depend>
<run_depend>message_runtime</run_depend>
Compile Code
Go to your catkin workspace and run catkin_make
cd catkin_ws
catkin_make
Verify Data Type Exist
rosmsg show Vector
Stop Topic
rosnode kill /cmd_move
All the details for this can be found in this video.