ROS Service
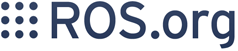
ROS Service
A service is a node that must run and you must wait until it has completed.
Commands
rosservice list
rosservice info <service name> # Node, URI, Type and Args
rossrv show <service name> # Args and return type
rosservice call <service name> <args> # "{}" is empty arg
Python Call ROS Service
import rospy
from gazebo_msgs.srv import DeleteModel, DeleteModelRequest
import sys
rospy.init_node('service_client')
rospy.wait_for_service('/gazebo/delete_model') # Wait for the service client to be running
delete_model_service = rospy.ServiceProxy('/gazebo/delete_model', DeleteModel) # Create the connection to the service
kk = DeleteRequest()
kk.model_name = "bowl_1" # Create an Arg for the service
result = delete_model_service(kk)
print(result)
Python Create an ROS Service
import rospy
from std_srvs.srv import Empty, EmptyResponse
def my_callback(request):
print "My_callback has been called"
return EmptyResponse()
#return MyServiceResponse(len(request.words.split()))
rospy.init_node('service_server')
my_service = rospy.Service('/my_service', Empty, my_callback)
rospy.spin() # maintain the service open
Call Service
rosservice call /my_service "{}"
Create a Catkin Service Project
Create Project
roscd
cd ../src/
catkin_create_pkg my_custom_srv_msg_pkg rospy
roscd my_custom_srv_msg_pkg
mkdir srv
vim srv/MyCustomServiceMessage.srv
Create Service File
Service file containing the arguments and return type.
float64 radius
int32 repititions
---
bool success
Modify CMakeList.txt and Package.xml
CMakeList.txt
find_package(catkin REQUIRED COMPONENTS
std_msgs
message_generation
)
add_service_files(
FILES
MyCustomServiceMessage.srv
)
catkin_package(
CATKIN_DEPENDS
rospy
)
Uncomment area
generate_messages(
DEPENDENCIES
std_msgs
)
Package.xml
Add Lines
<build_depend>message_generation</build_depend>
<run_depend>message_runtime</run_depend>
Build Project
roscd;cd ..
catkin_make
source devel/setup.bash
Test Project
rossrv list | grep MyCustomServiceMessage
rossrv show my_custom_srv_msg_pkg/MyCustomServiceMessage
More Details
Read other posts